在项目中,通常需要自己定义一些异常,以便更好的来管理我们的业务。通常来说,需要有一个我们自己的异常抽象,一个通用的异常类,以及一些特定条件下的异常类。如下所示:
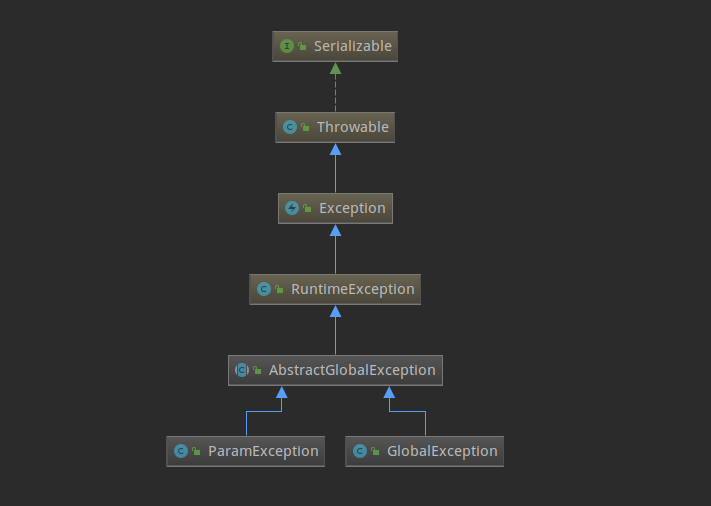
自定义异常抽象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package com.lazycece.sbac.exception.exception;
public abstract class AbstractGlobalException extends RuntimeException { public AbstractGlobalException() { }
public AbstractGlobalException(String message) { super(message); }
public AbstractGlobalException(String message, Throwable cause) { super(message, cause); }
public AbstractGlobalException(Throwable cause) { super(cause); }
abstract public int getCode(); }
|
自定义通用异常
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package com.lazycece.sbac.exception.exception;
public class GlobalException extends AbstractGlobalException {
private Integer code;
public GlobalException(String message, Integer code) { super(message); this.code = code; }
public GlobalException(String message, Throwable cause, Integer code) { super(message, cause); this.code = code; }
public GlobalException(Throwable cause, Integer code) { super(cause); this.code = code; }
@Override public int getCode() { return this.code; } }
|
特定域异常
特定域方面的异常通常随着业务的情况分为不同的类,这里以在业务层的参数校验的异常为例,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.lazycece.sbac.exception.exception;
import com.lazycece.sbac.exception.response.ResCode;
public class ParamException extends AbstractGlobalException { public ParamException() { }
public ParamException(String message) { super(message); }
public ParamException(String message, Throwable cause) { super(message, cause); }
@Override public int getCode() { return ResCode.PARAM_ERROR; } }
|
案例源码
案例源码地址:https://github.com/lazycece/springboot-actual-combat/tree/master/springboot-ac-exception